
项目介绍
近年来,信息化管理行业的不断兴起,使得人们的日常生活越来越离不开计算机和互联网技术。首先,根据收集到的用户需求分析,对设计系统有一个初步的认识与了解,确定校园失物招领网站的总体功能模块。然后,详细设计系统的主要功能模块,通过数据库设计过程将相关的数据信息存储到数据库中,再通过使用关键的开发工具,如IDEA开发平台、AJAX技术等,编码设计相关的功能模块。接着,主要采用功能测试的方式对系统进行测试,找出系统在运行过程中存在的问题,以及解决问题的方法,不断地改进和完善系统的设计。最后,总结本文介绍的系统的设计和实现过程,并且针对于系统的开发提出未来的展望工作。本系统的研发具有重大的意义,在安全性方面,用户使用浏览器访问网站时,采用注册和密码等相关的保护措施,提高系统的可靠性,维护用户的个人信息和财产的安全。在方便性方面,促进了校园失物招领网站的信息化建设,极大的方便了相关的工作人员对校园失物招领网站信息进行管理。
系统展示

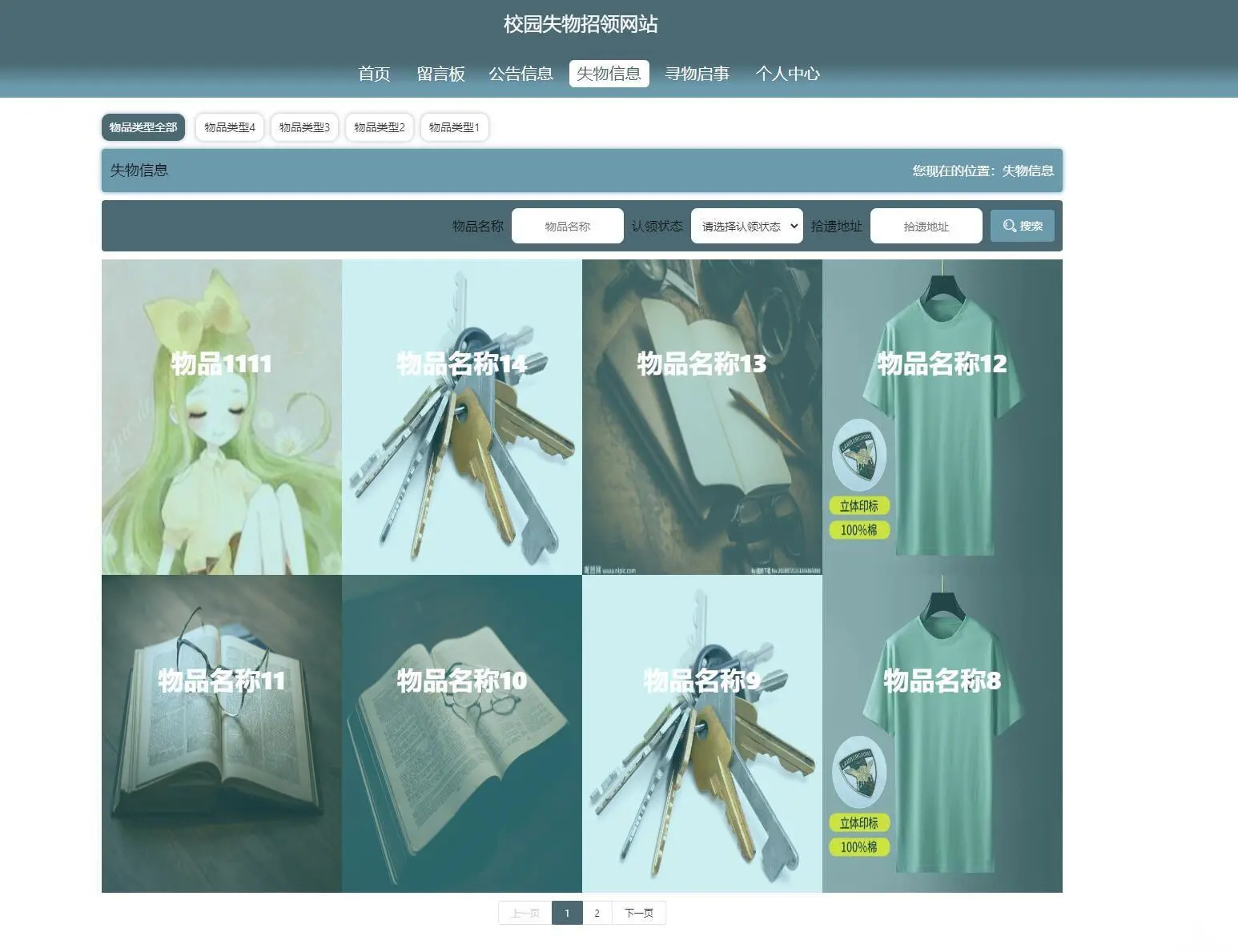
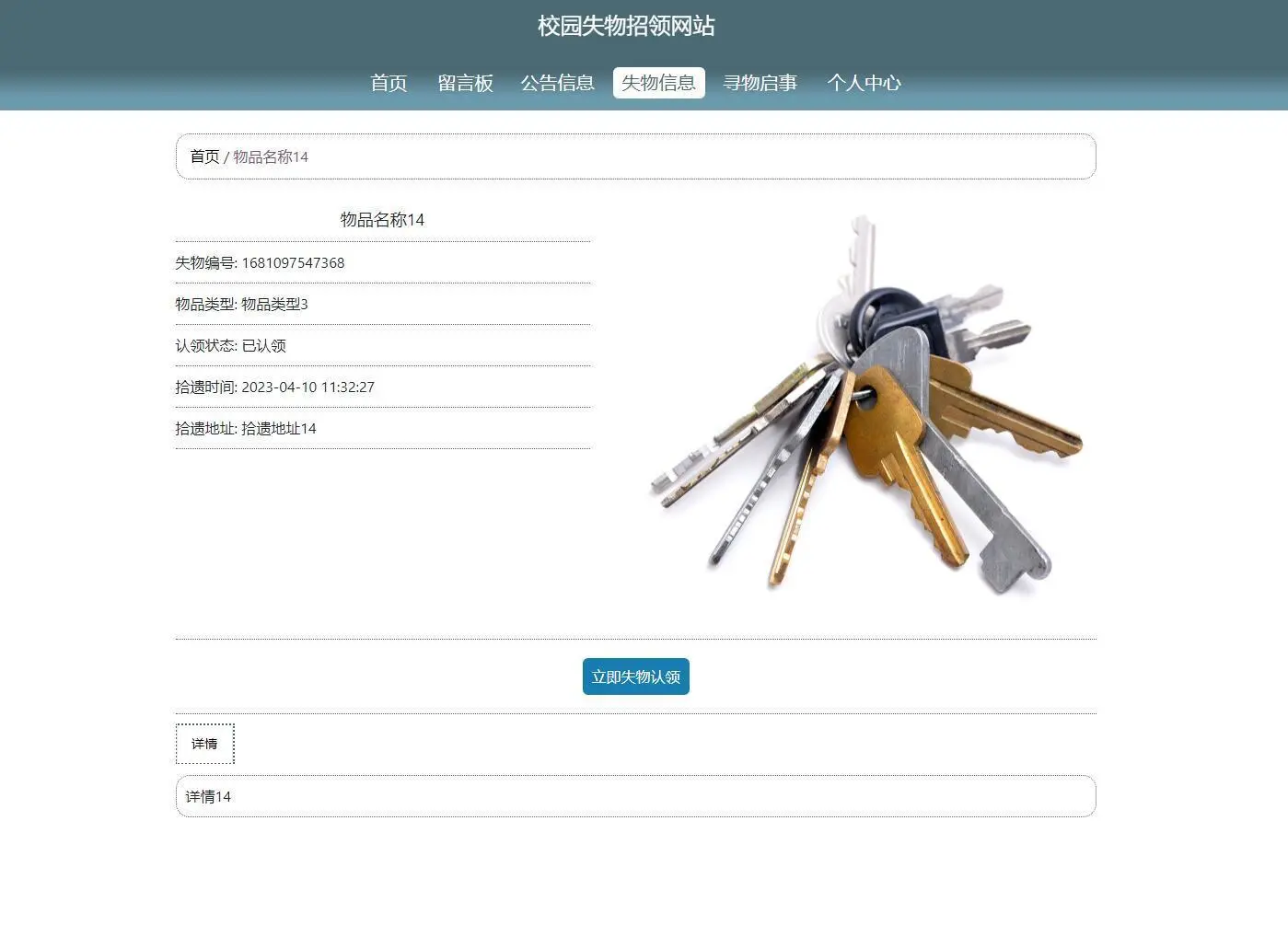

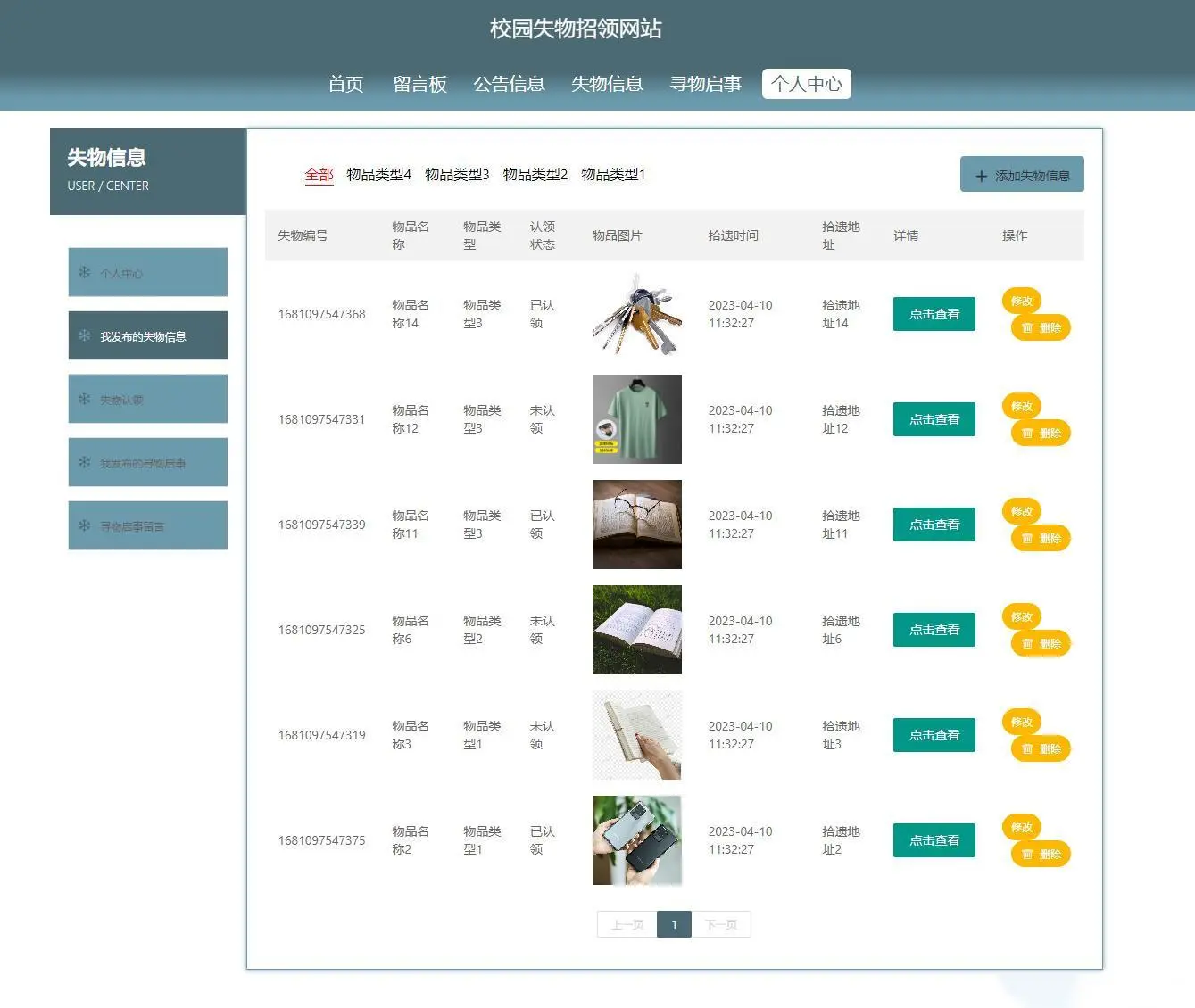
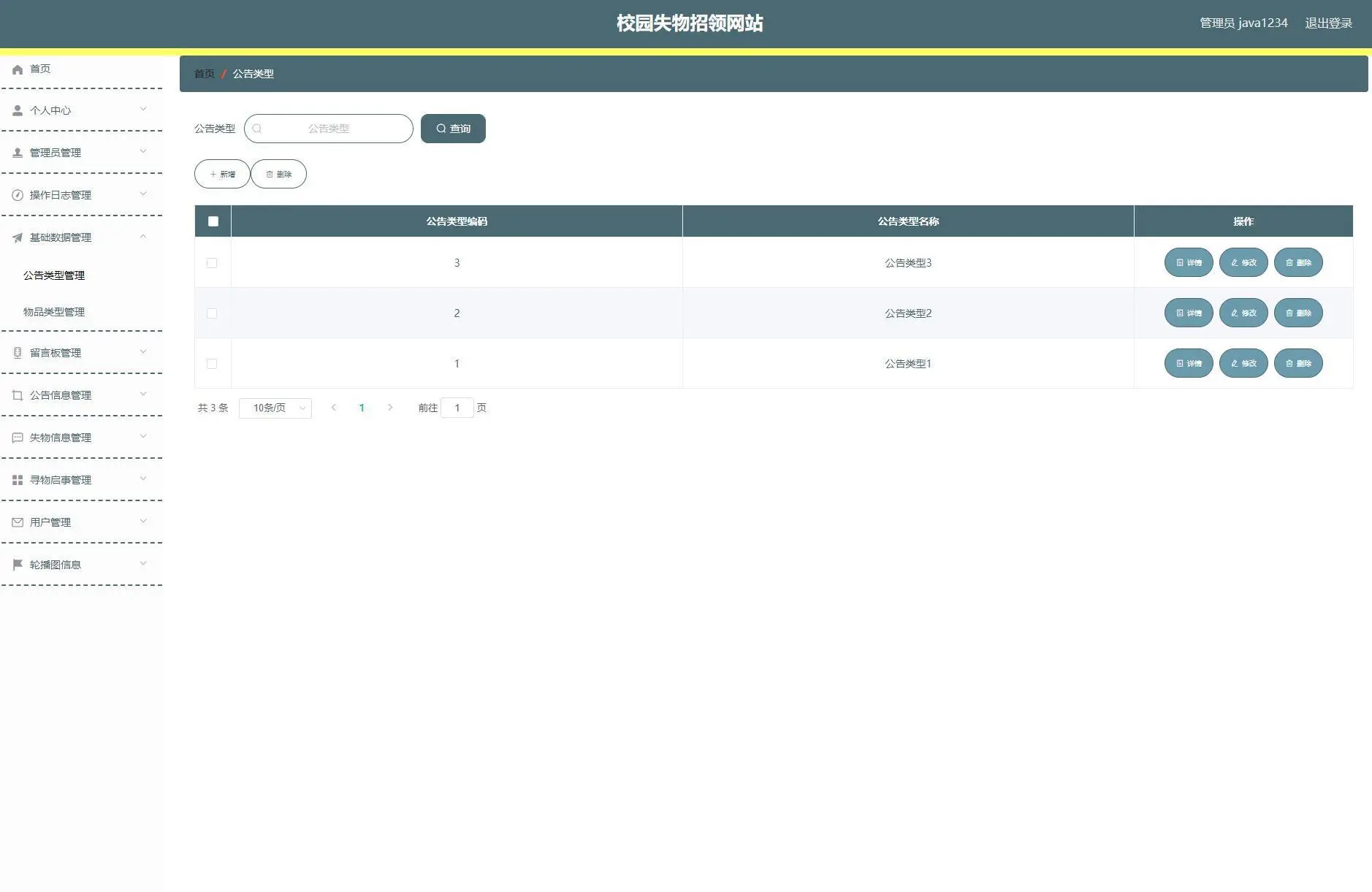
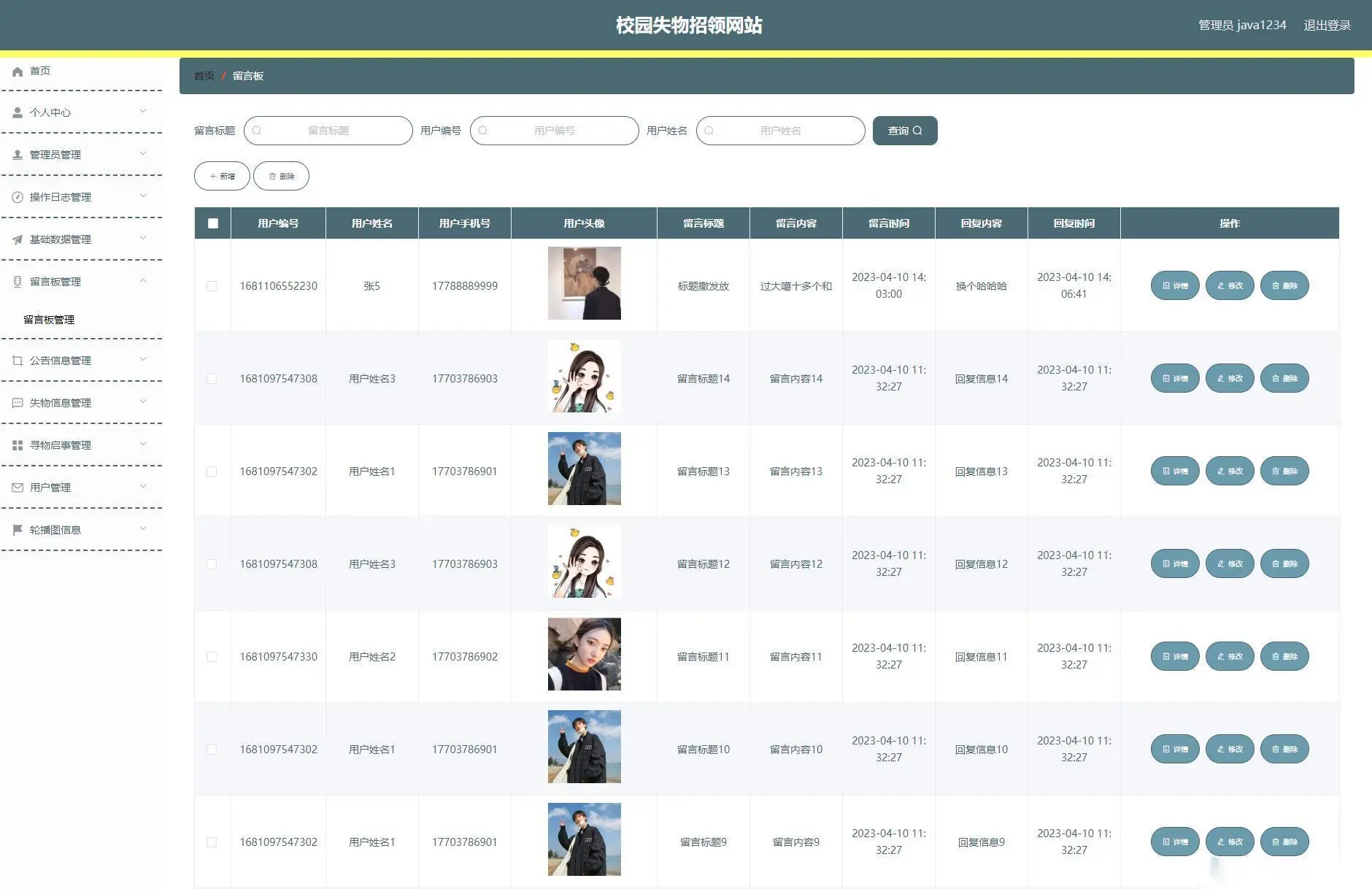
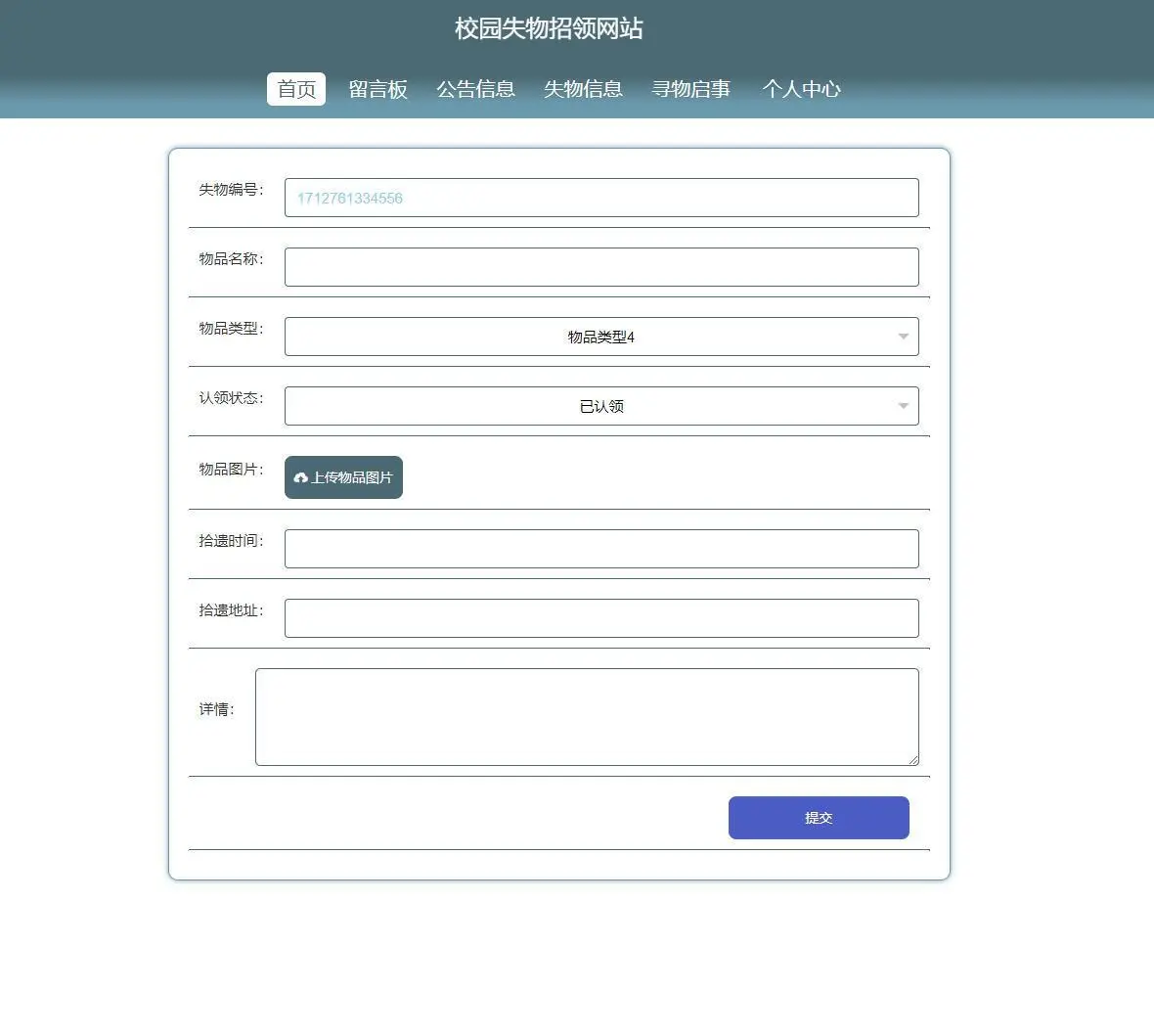
部分代码
package com.controller;
import java.io.File;
import java.math.BigDecimal;
import java.net.URL;
import java.text.SimpleDateFormat;
import com.alibaba.fastjson.JSONObject;
import java.util.*;
import org.springframework.beans.BeanUtils;
import javax.servlet.http.HttpServletRequest;
import org.springframework.web.context.ContextLoader;
import javax.servlet.ServletContext;
import com.service.TokenService;
import com.utils.*;
import java.lang.reflect.InvocationTargetException;
import com.service.DictionaryService;
import org.apache.commons.lang3.StringUtils;
import com.annotation.IgnoreAuth;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.entity.*;
import com.entity.view.*;
import com.service.*;
import com.utils.PageUtils;
import com.utils.R;
import com.alibaba.fastjson.*;
/**
* 用户
* 后端接口
* @author
* @email
*/
@RestController
@Controller
@RequestMapping("/yonghu")
public class YonghuController {
private static final Logger logger = LoggerFactory.getLogger(YonghuController.class);
private static final String TABLE_NAME = "yonghu";
@Autowired
private YonghuService yonghuService;
@Autowired
private TokenService tokenService;
@Autowired
private CaozuorizhiService caozuorizhiService;//操作日志
@Autowired
private DictionaryService dictionaryService;//字典
@Autowired
private LiuyanService liuyanService;//留言板
@Autowired
private NewsService newsService;//公告信息
@Autowired
private ShiwuzhaolingService shiwuzhaolingService;//失物信息
@Autowired
private ShiwuzhaolingYuyueService shiwuzhaolingYuyueService;//失物认领
@Autowired
private XunwuqishiService xunwuqishiService;//寻物启事
@Autowired
private XunwuqishiLiuyanService xunwuqishiLiuyanService;//寻物启事留言
@Autowired
private UsersService usersService;//管理员
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params, HttpServletRequest request){
logger.debug("page方法:,,Controller:{},,params:{}",this.getClass().getName(),JSONObject.toJSONString(params));
String role = String.valueOf(request.getSession().getAttribute("role"));
if(false)
return R.error(511,"永不会进入");
else if("用户".equals(role))
params.put("yonghuId",request.getSession().getAttribute("userId"));
CommonUtil.checkMap(params);
PageUtils page = yonghuService.queryPage(params);
//字典表数据转换
List<YonghuView> list =(List<YonghuView>)page.getList();
for(YonghuView c:list){
//修改对应字典表字段
dictionaryService.dictionaryConvert(c, request);
}
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"列表查询",list.toString());
return R.ok().put("data", page);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id, HttpServletRequest request){
logger.debug("info方法:,,Controller:{},,id:{}",this.getClass().getName(),id);
YonghuEntity yonghu = yonghuService.selectById(id);
if(yonghu !=null){
//entity转view
YonghuView view = new YonghuView();
BeanUtils.copyProperties( yonghu , view );//把实体数据重构到view中
//修改对应字典表字段
dictionaryService.dictionaryConvert(view, request);
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"单条数据查看",view.toString());
return R.ok().put("data", view);
}else {
return R.error(511,"查不到数据");
}
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
logger.debug("save方法:,,Controller:{},,yonghu:{}",this.getClass().getName(),yonghu.toString());
String role = String.valueOf(request.getSession().getAttribute("role"));
if(false)
return R.error(511,"永远不会进入");
Wrapper<YonghuEntity> queryWrapper = new EntityWrapper<YonghuEntity>()
.eq("username", yonghu.getUsername())
.or()
.eq("yonghu_phone", yonghu.getYonghuPhone())
.or()
.eq("yonghu_id_number", yonghu.getYonghuIdNumber())
;
logger.info("sql语句:"+queryWrapper.getSqlSegment());
YonghuEntity yonghuEntity = yonghuService.selectOne(queryWrapper);
if(yonghuEntity==null){
yonghu.setCreateTime(new Date());
yonghu.setPassword("123456");
yonghuService.insert(yonghu);
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"新增",yonghu.toString());
return R.ok();
}else {
return R.error(511,"账户或者用户手机号或者用户身份证号已经被使用");
}
}
/**
* 后端修改
*/
@RequestMapping("/update")
public R update(@RequestBody YonghuEntity yonghu, HttpServletRequest request) throws NoSuchFieldException, ClassNotFoundException, IllegalAccessException, InstantiationException {
logger.debug("update方法:,,Controller:{},,yonghu:{}",this.getClass().getName(),yonghu.toString());
YonghuEntity oldYonghuEntity = yonghuService.selectById(yonghu.getId());//查询原先数据
String role = String.valueOf(request.getSession().getAttribute("role"));
// if(false)
// return R.error(511,"永远不会进入");
if("".equals(yonghu.getYonghuPhoto()) || "null".equals(yonghu.getYonghuPhoto())){
yonghu.setYonghuPhoto(null);
}
yonghuService.updateById(yonghu);//根据id更新
List<String> strings = caozuorizhiService.clazzDiff(yonghu, oldYonghuEntity, request,new String[]{"updateTime"});
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"修改",strings.toString());
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Integer[] ids, HttpServletRequest request){
logger.debug("delete:,,Controller:{},,ids:{}",this.getClass().getName(),ids.toString());
List<YonghuEntity> oldYonghuList =yonghuService.selectBatchIds(Arrays.asList(ids));//要删除的数据
yonghuService.deleteBatchIds(Arrays.asList(ids));
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"删除",oldYonghuList.toString());
return R.ok();
}
/**
* 批量上传
*/
@RequestMapping("/batchInsert")
public R save( String fileName, HttpServletRequest request){
logger.debug("batchInsert方法:,,Controller:{},,fileName:{}",this.getClass().getName(),fileName);
Integer yonghuId = Integer.valueOf(String.valueOf(request.getSession().getAttribute("userId")));
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
//.eq("time", new SimpleDateFormat("yyyy-MM-dd").format(new Date()))
try {
List<YonghuEntity> yonghuList = new ArrayList<>();//上传的东西
Map<String, List<String>> seachFields= new HashMap<>();//要查询的字段
Date date = new Date();
int lastIndexOf = fileName.lastIndexOf(".");
if(lastIndexOf == -1){
return R.error(511,"该文件没有后缀");
}else{
String suffix = fileName.substring(lastIndexOf);
if(!".xls".equals(suffix)){
return R.error(511,"只支持后缀为xls的excel文件");
}else{
URL resource = this.getClass().getClassLoader().getResource("static/upload/" + fileName);//获取文件路径
File file = new File(resource.getFile());
if(!file.exists()){
return R.error(511,"找不到上传文件,请联系管理员");
}else{
List<List<String>> dataList = PoiUtil.poiImport(file.getPath());//读取xls文件
dataList.remove(0);//删除第一行,因为第一行是提示
for(List<String> data:dataList){
//循环
YonghuEntity yonghuEntity = new YonghuEntity();
// yonghuEntity.setUsername(data.get(0)); //账户 要改的
// yonghuEntity.setPassword("123456");//密码
// yonghuEntity.setYonghuUuidNumber(data.get(0)); //用户编号 要改的
// yonghuEntity.setYonghuName(data.get(0)); //用户姓名 要改的
// yonghuEntity.setYonghuPhone(data.get(0)); //用户手机号 要改的
// yonghuEntity.setYonghuIdNumber(data.get(0)); //用户身份证号 要改的
// yonghuEntity.setYonghuPhoto("");//详情和图片
// yonghuEntity.setSexTypes(Integer.valueOf(data.get(0))); //性别 要改的
// yonghuEntity.setYonghuEmail(data.get(0)); //用户邮箱 要改的
// yonghuEntity.setJinyongTypes(Integer.valueOf(data.get(0))); //账户状态 要改的
// yonghuEntity.setCreateTime(date);//时间
yonghuList.add(yonghuEntity);
//把要查询是否重复的字段放入map中
//账户
if(seachFields.containsKey("username")){
List<String> username = seachFields.get("username");
username.add(data.get(0));//要改的
}else{
List<String> username = new ArrayList<>();
username.add(data.get(0));//要改的
seachFields.put("username",username);
}
//用户编号
if(seachFields.containsKey("yonghuUuidNumber")){
List<String> yonghuUuidNumber = seachFields.get("yonghuUuidNumber");
yonghuUuidNumber.add(data.get(0));//要改的
}else{
List<String> yonghuUuidNumber = new ArrayList<>();
yonghuUuidNumber.add(data.get(0));//要改的
seachFields.put("yonghuUuidNumber",yonghuUuidNumber);
}
//用户手机号
if(seachFields.containsKey("yonghuPhone")){
List<String> yonghuPhone = seachFields.get("yonghuPhone");
yonghuPhone.add(data.get(0));//要改的
}else{
List<String> yonghuPhone = new ArrayList<>();
yonghuPhone.add(data.get(0));//要改的
seachFields.put("yonghuPhone",yonghuPhone);
}
//用户身份证号
if(seachFields.containsKey("yonghuIdNumber")){
List<String> yonghuIdNumber = seachFields.get("yonghuIdNumber");
yonghuIdNumber.add(data.get(0));//要改的
}else{
List<String> yonghuIdNumber = new ArrayList<>();
yonghuIdNumber.add(data.get(0));//要改的
seachFields.put("yonghuIdNumber",yonghuIdNumber);
}
}
//查询是否重复
//账户
List<YonghuEntity> yonghuEntities_username = yonghuService.selectList(new EntityWrapper<YonghuEntity>().in("username", seachFields.get("username")));
if(yonghuEntities_username.size() >0 ){
ArrayList<String> repeatFields = new ArrayList<>();
for(YonghuEntity s:yonghuEntities_username){
repeatFields.add(s.getUsername());
}
return R.error(511,"数据库的该表中的 [账户] 字段已经存在 存在数据为:"+repeatFields.toString());
}
//用户编号
List<YonghuEntity> yonghuEntities_yonghuUuidNumber = yonghuService.selectList(new EntityWrapper<YonghuEntity>().in("yonghu_uuid_number", seachFields.get("yonghuUuidNumber")));
if(yonghuEntities_yonghuUuidNumber.size() >0 ){
ArrayList<String> repeatFields = new ArrayList<>();
for(YonghuEntity s:yonghuEntities_yonghuUuidNumber){
repeatFields.add(s.getYonghuUuidNumber());
}
return R.error(511,"数据库的该表中的 [用户编号] 字段已经存在 存在数据为:"+repeatFields.toString());
}
//用户手机号
List<YonghuEntity> yonghuEntities_yonghuPhone = yonghuService.selectList(new EntityWrapper<YonghuEntity>().in("yonghu_phone", seachFields.get("yonghuPhone")));
if(yonghuEntities_yonghuPhone.size() >0 ){
ArrayList<String> repeatFields = new ArrayList<>();
for(YonghuEntity s:yonghuEntities_yonghuPhone){
repeatFields.add(s.getYonghuPhone());
}
return R.error(511,"数据库的该表中的 [用户手机号] 字段已经存在 存在数据为:"+repeatFields.toString());
}
//用户身份证号
List<YonghuEntity> yonghuEntities_yonghuIdNumber = yonghuService.selectList(new EntityWrapper<YonghuEntity>().in("yonghu_id_number", seachFields.get("yonghuIdNumber")));
if(yonghuEntities_yonghuIdNumber.size() >0 ){
ArrayList<String> repeatFields = new ArrayList<>();
for(YonghuEntity s:yonghuEntities_yonghuIdNumber){
repeatFields.add(s.getYonghuIdNumber());
}
return R.error(511,"数据库的该表中的 [用户身份证号] 字段已经存在 存在数据为:"+repeatFields.toString());
}
yonghuService.insertBatch(yonghuList);
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"批量新增",yonghuList.toString());
return R.ok();
}
}
}
}catch (Exception e){
e.printStackTrace();
return R.error(511,"批量插入数据异常,请联系管理员");
}
}
/**
* 登录
*/
@IgnoreAuth
@RequestMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
YonghuEntity yonghu = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("username", username));
if(yonghu==null || !yonghu.getPassword().equals(password))
return R.error("账号或密码不正确");
else if(yonghu.getJinyongTypes() != 1)
return R.error("账户已被禁用");
String token = tokenService.generateToken(yonghu.getId(),username, "yonghu", "用户");
R r = R.ok();
r.put("token", token);
r.put("role","用户");
r.put("username",yonghu.getYonghuName());
r.put("tableName","yonghu");
r.put("userId",yonghu.getId());
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"登录",yonghu.toString());
return r;
}
/**
* 注册
*/
@IgnoreAuth
@PostMapping(value = "/register")
public R register(@RequestBody YonghuEntity yonghu, HttpServletRequest request) {
// ValidatorUtils.validateEntity(user);
Wrapper<YonghuEntity> queryWrapper = new EntityWrapper<YonghuEntity>()
.eq("username", yonghu.getUsername())
.or()
.eq("yonghu_phone", yonghu.getYonghuPhone())
.or()
.eq("yonghu_id_number", yonghu.getYonghuIdNumber())
;
YonghuEntity yonghuEntity = yonghuService.selectOne(queryWrapper);
if(yonghuEntity != null)
return R.error("账户或者用户手机号或者用户身份证号已经被使用");
yonghu.setYonghuUuidNumber(String.valueOf(new Date().getTime()));
yonghu.setJinyongTypes(1);//启用
yonghu.setCreateTime(new Date());
yonghuService.insert(yonghu);
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"注册",yonghu.toString());
return R.ok();
}
/**
* 重置密码
*/
@GetMapping(value = "/resetPassword")
public R resetPassword(Integer id, HttpServletRequest request) {
YonghuEntity yonghu = yonghuService.selectById(id);
yonghu.setPassword("123456");
yonghuService.updateById(yonghu);
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"重置密码",yonghu.toString());
return R.ok();
}
/**
* 修改密码
*/
@GetMapping(value = "/updatePassword")
public R updatePassword(String oldPassword, String newPassword, HttpServletRequest request) {
YonghuEntity yonghu = yonghuService.selectById((Integer)request.getSession().getAttribute("userId"));
if(newPassword == null){
return R.error("新密码不能为空") ;
}
if(!oldPassword.equals(yonghu.getPassword())){
return R.error("原密码输入错误");
}
if(newPassword.equals(yonghu.getPassword())){
return R.error("新密码不能和原密码一致") ;
}
yonghu.setPassword(newPassword);
yonghuService.updateById(yonghu);
return R.ok();
}
/**
* 忘记密码
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request) {
YonghuEntity yonghu = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("username", username));
if(yonghu!=null){
yonghu.setPassword("123456");
yonghuService.updateById(yonghu);
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"忘记密码",yonghu.toString());
return R.ok();
}else{
return R.error("账号不存在");
}
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrYonghu(HttpServletRequest request){
Integer id = (Integer)request.getSession().getAttribute("userId");
YonghuEntity yonghu = yonghuService.selectById(id);
if(yonghu !=null){
//entity转view
YonghuView view = new YonghuView();
BeanUtils.copyProperties( yonghu , view );//把实体数据重构到view中
//修改对应字典表字段
dictionaryService.dictionaryConvert(view, request);
return R.ok().put("data", view);
}else {
return R.error(511,"查不到数据");
}
}
/**
* 退出
*/
@GetMapping(value = "logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params, HttpServletRequest request){
logger.debug("list方法:,,Controller:{},,params:{}",this.getClass().getName(),JSONObject.toJSONString(params));
CommonUtil.checkMap(params);
PageUtils page = yonghuService.queryPage(params);
//字典表数据转换
List<YonghuView> list =(List<YonghuView>)page.getList();
for(YonghuView c:list)
dictionaryService.dictionaryConvert(c, request); //修改对应字典表字段
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"列表查询",list.toString());
return R.ok().put("data", page);
}
/**
* 前端详情
*/
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id, HttpServletRequest request){
logger.debug("detail方法:,,Controller:{},,id:{}",this.getClass().getName(),id);
YonghuEntity yonghu = yonghuService.selectById(id);
if(yonghu !=null){
//entity转view
YonghuView view = new YonghuView();
BeanUtils.copyProperties( yonghu , view );//把实体数据重构到view中
//修改对应字典表字段
dictionaryService.dictionaryConvert(view, request);
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"单条数据查看",view.toString());
return R.ok().put("data", view);
}else {
return R.error(511,"查不到数据");
}
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
logger.debug("add方法:,,Controller:{},,yonghu:{}",this.getClass().getName(),yonghu.toString());
Wrapper<YonghuEntity> queryWrapper = new EntityWrapper<YonghuEntity>()
.eq("username", yonghu.getUsername())
.or()
.eq("yonghu_phone", yonghu.getYonghuPhone())
.or()
.eq("yonghu_id_number", yonghu.getYonghuIdNumber())
// .notIn("yonghu_types", new Integer[]{102})
;
logger.info("sql语句:"+queryWrapper.getSqlSegment());
YonghuEntity yonghuEntity = yonghuService.selectOne(queryWrapper);
if(yonghuEntity==null){
yonghu.setCreateTime(new Date());
yonghu.setPassword("123456");
yonghuService.insert(yonghu);
caozuorizhiService.insertCaozuorizhi(String.valueOf(request.getSession().getAttribute("role")),TABLE_NAME,String.valueOf(request.getSession().getAttribute("username")),"前台新增",yonghu.toString());
return R.ok();
}else {
return R.error(511,"账户或者用户手机号或者用户身份证号已经被使用");
}
}
}
<template>
<div>
<div class="container loginIn" style="backgroundImage: url(/xiaoyuanshiwu/img/back-img-bg.jpg)">
<div :class="2 == 1 ? 'left' : 2 == 2 ? 'left center' : 'left right'" style="backgroundColor: rgba(237, 237, 237, 0.17)">
<el-form class="login-form" label-position="left" :label-width="1 == 3 ? '56px' : '0px'">
<div class="title-container"><h3 class="title" style="color: var(--publicMainColor)">校园失物招领网站</h3></div>
<el-form-item :label="1 == 3 ? '用户名' : ''" :class="'style'+1">
<span v-if="1 != 3" class="svg-container" style="color:rgba(136, 154, 164, 1);line-height:44px"><svg-icon icon-class="user" /></span>
<el-input placeholder="请输入用户名" name="username" type="text" v-model="rulesForm.username" />
</el-form-item>
<el-form-item :label="1 == 3 ? '密码' : ''" :class="'style'+1">
<span v-if="1 != 3" class="svg-container" style="color:rgba(136, 154, 164, 1);line-height:44px"><svg-icon icon-class="password" /></span>
<el-input placeholder="请输入密码" name="password" type="password" v-model="rulesForm.password" />
</el-form-item>
<el-form-item v-if="0 == '1'" class="code" :label="1 == 3 ? '验证码' : ''" :class="'style'+1">
<span v-if="1 != 3" class="svg-container" style="color:rgba(136, 154, 164, 1);line-height:44px"><svg-icon icon-class="code" /></span>
<el-input placeholder="请输入验证码" name="code" type="text" v-model="rulesForm.code" />
<div class="getCodeBt" @click="getRandCode(4)" style="height:44px;line-height:44px">
<span v-for="(item, index) in codes" :key="index" :style="{color:item.color,transform:item.rotate,fontSize:item.size}">{{ item.num }}</span>
</div>
</el-form-item>
<el-form-item v-if="roleOptions.length>1" label="角色" prop="loginInRole" class="role" style="display: flex;align-items: center;">
<el-radio
@change="menuChange"
v-for="item in roleOptions"
v-bind:key="item.value"
v-model="rulesForm.role"
:label="item.value"
>{{item.key}}</el-radio>
</el-form-item>
<el-button type="primary" @click="login()" class="loginInBt" style="padding:0;font-size:16px;border-radius:4px;height:44px;line-height:44px;width:100%;backgroundColor:var(--publicMainColor); borderColor:var(--publicMainColor); color:rgba(255, 255, 255, 1)">{{'1' == '1' ? '登录' : 'login'}}</el-button> <el-form-item class="setting">
<!-- <div class="register" @click="register('yonghu')">用户注册</div>-->
<p align=center style="padding-top:10px"><a href="http://www.java1234.com/a/bysj/javaweb/" target='_blank'><font color=red>Java1234收藏整理</font></a></p>
</el-form-item>
</el-form>
</div>
<!--
<el-form-item v-if="0 == '1'" class="code" :label="3 == 3 ? '验证码' : ''" :class="'style'+3">
<span v-if="3 != 3" class="svg-container" style="color:rgba(136, 154, 164, 1);line-height:46px"><svg-icon icon-class="code" /></span>
<el-input placeholder="请输入验证码" name="code" type="text" v-model="rulesForm.code" />
<div class="getCodeBt" @click="getRandCode(4)" style="height:46px;line-height:46px">
<span v-for="(item, index) in codes" :key="index" :style="{color:item.color,transform:item.rotate,fontSize:item.size}">{{ item.num }}</span>
</div>
</el-form-item>
-->
</div>
</div>
</template>
<script>
import menu from "@/utils/menu";
export default {
data() {
return {
rulesForm: {
username: "",
password: "",
role: "",
code: '',
},
menus: [],
roleOptions: [],
tableName: "",
codes: [{
num: 1,
color: '#000',
rotate: '10deg',
size: '16px'
},{
num: 2,
color: '#000',
rotate: '10deg',
size: '16px'
},{
num: 3,
color: '#000',
rotate: '10deg',
size: '16px'
},{
num: 4,
color: '#000',
rotate: '10deg',
size: '16px'
}],
};
},
mounted() {
let menus = menu.list();
this.menus = menus;
for (let i = 0; i < this.menus.length; i++) {
if (this.menus[i].hasBackLogin=='是') {
let menuItem={};
menuItem["key"]=this.menus[i].roleName;
menuItem["value"]=this.menus[i].tableName;
this.roleOptions.push(menuItem);
}
}
},
created() {
this.getRandCode()
},
methods: {
menuChange(role){
},
register(tableName){
this.$storage.set("loginTable", tableName);
this.$router.push({path:'/register'})
},
// 登陆
login() {
let code = ''
for(let i in this.codes) {
code += this.codes[i].num
}
if ('0' == '1' && !this.rulesForm.code) {
this.$message.error("请输入验证码");
return;
}
if ('0' == '1' && this.rulesForm.code.toLowerCase() != code.toLowerCase()) {
this.$message.error("验证码输入有误");
this.getRandCode()
return;
}
if (!this.rulesForm.username) {
this.$message.error("请输入用户名");
return;
}
if (!this.rulesForm.password) {
this.$message.error("请输入密码");
return;
}
if(this.roleOptions.length>1) {
console.log("1")
if (!this.rulesForm.role) {
this.$message.error("请选择角色");
return;
}
let menus = this.menus;
for (let i = 0; i < menus.length; i++) {
if (menus[i].tableName == this.rulesForm.role) {
this.tableName = menus[i].tableName;
this.rulesForm.role = menus[i].roleName;
}
}
} else {
this.tableName = this.roleOptions[0].value;
this.rulesForm.role = this.roleOptions[0].key;
}
this.$http({
url: `${this.tableName}/login?username=${this.rulesForm.username}&password=${this.rulesForm.password}`,
method: "post"
}).then(({ data }) => {
if (data && data.code === 0) {
this.$storage.set("Token", data.token);
this.$storage.set("userId", data.userId);
this.$storage.set("role", this.rulesForm.role);
this.$storage.set("sessionTable", this.tableName);
this.$storage.set("adminName", this.rulesForm.username);
this.$router.replace({ path: "/index/" });
} else {
this.$message.error(data.msg);
}
});
},
getRandCode(len = 4){
this.randomString(len)
},
randomString(len = 4) {
let chars = [
"a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k",
"l", "m", "n", "o", "p", "q", "r", "s", "t", "u", "v",
"w", "x", "y", "z", "A", "B", "C", "D", "E", "F", "G",
"H", "I", "J", "K", "L", "M", "N", "O", "P", "Q", "R",
"S", "T", "U", "V", "W", "X", "Y", "Z", "0", "1", "2",
"2", "4", "5", "6", "7", "8", "9"
]
let colors = ["0", "1", "2","2", "4", "5", "6", "7", "8", "9", "a", "b", "c", "d", "e", "f"]
let sizes = ['14', '15', '16', '17', '18']
let output = [];
for (let i = 0; i < len; i++) {
// 随机验证码
let key = Math.floor(Math.random()*chars.length)
this.codes[i].num = chars[key]
// 随机验证码颜色
let code = '#'
for (let j = 0; j < 6; j++) {
let key = Math.floor(Math.random()*colors.length)
code += colors[key]
}
this.codes[i].color = code
// 随机验证码方向
let rotate = Math.floor(Math.random()*60)
let plus = Math.floor(Math.random()*2)
if(plus == 1) rotate = '-'+rotate
this.codes[i].rotate = 'rotate('+rotate+'deg)'
// 随机验证码字体大小
let size = Math.floor(Math.random()*sizes.length)
this.codes[i].size = sizes[size]+'px'
}
},
}
};
</script>
<style lang="scss" scoped>
.loginIn {
min-height: 100vh;
position: relative;
background-repeat: no-repeat;
background-position: center center;
background-size: cover;
.left {
position: absolute;
left: 0;
top: 0;
width: 360px;
height: 100%;
.login-form {
background-color: #fff;
width: 100%;
right: inherit;
padding: 0 12px;
box-sizing: border-box;
display: flex;
justify-content: center;
flex-direction: column;
}
.title-container {
text-align: center;
font-size: 24px;
.title {
margin: 20px 0;
}
}
.el-form-item {
position: relative;
.svg-container {
padding: 6px 5px 6px 15px;
color: #889aa4;
vertical-align: middle;
display: inline-block;
position: absolute;
left: 0;
top: 0;
z-index: 1;
padding: 0;
line-height: 40px;
width: 30px;
text-align: center;
}
.el-input {
display: inline-block;
height: 40px;
width: 100%;
& /deep/ input {
background: transparent;
border: 0px;
-webkit-appearance: none;
padding: 0 15px 0 30px;
color: var(--publicMainColor);
height: 40px;
}
}
}
}
.center {
position: absolute;
left: 50%;
top: 50%;
width: 360px;
transform: translate3d(-50%,-50%,0);
height: 446px;
border-radius: 8px;
}
.right {
position: absolute;
left: inherit;
right: 0;
top: 0;
width: 360px;
height: 100%;
}
.code {
.el-form-item__content {
position: relative;
.getCodeBt {
position: absolute;
right: 0;
top: 0;
line-height: 40px;
width: 100px;
background-color: rgba(51,51,51,0.4);
color: #fff;
text-align: center;
border-radius: 0 4px 4px 0;
height: 40px;
overflow: hidden;
span {
padding: 0 5px;
display: inline-block;
font-size: 16px;
font-weight: 600;
}
}
.el-input {
& /deep/ input {
padding: 0 130px 0 30px;
}
}
}
}
.setting {
& /deep/ .el-form-item__content {
padding: 0 15px;
box-sizing: border-box;
line-height: 32px;
height: 32px;
font-size: 14px;
color: #999;
margin: 0 !important;
.register {
float: left;
width: 50%;
}
.reset {
float: right;
width: 50%;
text-align: right;
}
}
}
.style2 {
padding-left: 30px;
.svg-container {
left: -30px !important;
}
.el-input {
& /deep/ input {
padding: 0 15px !important;
}
}
}
.code.style2, .code.style3 {
.el-input {
& /deep/ input {
padding: 0 115px 0 15px;
}
}
}
.style3 {
& /deep/ .el-form-item__label {
padding-right: 6px;
}
.el-input {
& /deep/ input {
padding: 0 15px !important;
}
}
}
.role {
& /deep/ .el-form-item__label {
width: 56px !important;
color: var(--publicMainColor);
}
& /deep/ .el-radio {
margin-right: 12px;
color: var(--publicMainColor);
}
}
}
</style>
源码下载
请在下方用积分兑换
感谢您的访问,Ctrl+D收藏本站吧。

© 版权声明
THE END
暂无评论内容